Introduce GiteaLocaleNumber custom element to handle number localization on pages. (#23861)
Follow #21429 & #22861 Use `<gitea-locale-number>` instead of backend `PrettyNumber`. All old `PrettyNumber` related functions are removed. A lot of code could be simplified. And some functions haven't been used for long time (dead code), so they are also removed by the way (eg: `SplitStringAtRuneN`, `Dedent`) This PR only tries to improve the `PrettyNumber` rendering problem, it doesn't touch the "plural" problem. Screenshot: 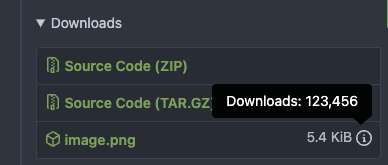 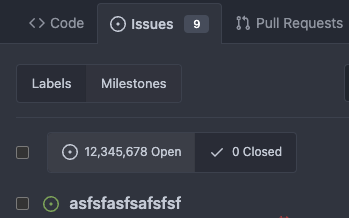
This commit is contained in:
parent
01d9466bfd
commit
19de52e0f4
24 changed files with 94 additions and 227 deletions
|
@ -1,20 +1,9 @@
|
|||
import {prettyNumber} from '../utils.js';
|
||||
|
||||
const {lang} = document.documentElement;
|
||||
const dateFormatter = new Intl.DateTimeFormat(lang, {year: 'numeric', month: 'long', day: 'numeric'});
|
||||
const shortDateFormatter = new Intl.DateTimeFormat(lang, {year: 'numeric', month: 'short', day: 'numeric'});
|
||||
const dateTimeFormatter = new Intl.DateTimeFormat(lang, {year: 'numeric', month: 'short', day: 'numeric', hour: 'numeric', minute: 'numeric', second: 'numeric'});
|
||||
|
||||
export function initFormattingReplacements() {
|
||||
// replace english formatted numbers with locale-specific separators
|
||||
for (const el of document.getElementsByClassName('js-pretty-number')) {
|
||||
const num = Number(el.getAttribute('data-value'));
|
||||
const formatted = prettyNumber(num, lang);
|
||||
if (formatted && formatted !== el.textContent) {
|
||||
el.textContent = formatted;
|
||||
}
|
||||
}
|
||||
|
||||
// for each <time></time> tag, if it has the data-format attribute, format
|
||||
// the text according to the user's chosen locale and formatter.
|
||||
formatAllTimeElements();
|
||||
|
|
|
@ -54,13 +54,6 @@ export function parseIssueHref(href) {
|
|||
return {owner, repo, type, index};
|
||||
}
|
||||
|
||||
// pretty-print a number using locale-specific separators, e.g. 1200 -> 1,200
|
||||
export function prettyNumber(num, locale = 'en-US') {
|
||||
if (typeof num !== 'number') return '';
|
||||
const {format} = new Intl.NumberFormat(locale);
|
||||
return format(num);
|
||||
}
|
||||
|
||||
// parse a URL, either relative '/path' or absolute 'https://localhost/path'
|
||||
export function parseUrl(str) {
|
||||
return new URL(str, str.startsWith('http') ? undefined : window.location.origin);
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
import {expect, test} from 'vitest';
|
||||
import {
|
||||
basename, extname, isObject, stripTags, joinPaths, parseIssueHref,
|
||||
prettyNumber, parseUrl, translateMonth, translateDay, blobToDataURI,
|
||||
parseUrl, translateMonth, translateDay, blobToDataURI,
|
||||
toAbsoluteUrl,
|
||||
} from './utils.js';
|
||||
|
||||
|
@ -84,17 +84,6 @@ test('parseIssueHref', () => {
|
|||
expect(parseIssueHref('')).toEqual({owner: undefined, repo: undefined, type: undefined, index: undefined});
|
||||
});
|
||||
|
||||
test('prettyNumber', () => {
|
||||
expect(prettyNumber()).toEqual('');
|
||||
expect(prettyNumber(null)).toEqual('');
|
||||
expect(prettyNumber(undefined)).toEqual('');
|
||||
expect(prettyNumber('1200')).toEqual('');
|
||||
expect(prettyNumber(12345678, 'en-US')).toEqual('12,345,678');
|
||||
expect(prettyNumber(12345678, 'de-DE')).toEqual('12.345.678');
|
||||
expect(prettyNumber(12345678, 'be-BE')).toEqual('12 345 678');
|
||||
expect(prettyNumber(12345678, 'hi-IN')).toEqual('1,23,45,678');
|
||||
});
|
||||
|
||||
test('parseUrl', () => {
|
||||
expect(parseUrl('').pathname).toEqual('/');
|
||||
expect(parseUrl('/path').pathname).toEqual('/path');
|
||||
|
|
20
web_src/js/webcomponents/GiteaLocaleNumber.js
Normal file
20
web_src/js/webcomponents/GiteaLocaleNumber.js
Normal file
|
@ -0,0 +1,20 @@
|
|||
// Convert a number to a locale string by data-number attribute.
|
||||
// Or add a tooltip by data-number-in-tooltip attribute. JSON: {message: "count: %s", number: 123}
|
||||
window.customElements.define('gitea-locale-number', class extends HTMLElement {
|
||||
connectedCallback() {
|
||||
// ideally, the number locale formatting and plural processing should be done by backend with translation strings.
|
||||
// if we have complete backend locale support (eg: Golang "x/text" package), we can drop this component.
|
||||
const number = this.getAttribute('data-number');
|
||||
if (number) {
|
||||
this.attachShadow({mode: 'open'});
|
||||
this.shadowRoot.textContent = new Intl.NumberFormat().format(Number(number));
|
||||
}
|
||||
const numberInTooltip = this.getAttribute('data-number-in-tooltip');
|
||||
if (numberInTooltip) {
|
||||
// TODO: only 2 usages of this, we can replace it with Golang's "x/text/number" package in the future
|
||||
const {message, number} = JSON.parse(numberInTooltip);
|
||||
const tooltipContent = message.replace(/%[ds]/, new Intl.NumberFormat().format(Number(number)));
|
||||
this.setAttribute('data-tooltip-content', tooltipContent);
|
||||
}
|
||||
}
|
||||
});
|
|
@ -1,6 +1,4 @@
|
|||
import '@webcomponents/custom-elements'; // automatically adds custom elements for older browsers that don't support it
|
||||
|
||||
// this is a Gitea's private HTML component, it converts an absolute or relative URL to an absolute URL with the current origin
|
||||
// Convert an absolute or relative URL to an absolute URL with the current origin
|
||||
window.customElements.define('gitea-origin-url', class extends HTMLElement {
|
||||
connectedCallback() {
|
||||
const urlStr = this.getAttribute('data-url');
|
||||
|
|
|
@ -15,5 +15,4 @@ https://developer.mozilla.org/en-US/docs/Web/Web_Components
|
|||
|
||||
There are still some components that are not migrated to web components yet:
|
||||
|
||||
* `<span class="js-pretty-number">`
|
||||
* `<time data-format>`
|
||||
|
|
3
web_src/js/webcomponents/webcomponents.js
Normal file
3
web_src/js/webcomponents/webcomponents.js
Normal file
|
@ -0,0 +1,3 @@
|
|||
import '@webcomponents/custom-elements'; // polyfill for some browsers like Pale Moon
|
||||
import './GiteaLocaleNumber.js';
|
||||
import './GiteaOriginUrl.js';
|
Loading…
Add table
Add a link
Reference in a new issue